QML Mod - 4 channel support on param reset upon song load
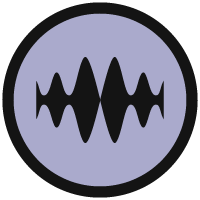
I've been getting some success resetting stem parameters on a deck when loading a song. My code works for deck 1 and 2, but I can't get it to work for deck 3 and 4.
I tweaked the resetFocusedStemDeckVolumeAndFilter function using the structure shown below:
function resetFocusedStemDeckVolumeAndFilter(deckId) { var defaultVolume = 1.0; if (deckId == topDeckId) { // Set all volumes to maximum topStemVolume1.value = defaultVolume; topStemVolume2.value = defaultVolume; topStemVolume3.value = defaultVolume; topStemVolume4.value = defaultVolume; } else { // Set all volumes to maximum bottomStemVolume1.value = defaultVolume; bottomStemVolume2.value = defaultVolume; bottomStemVolume3.value = defaultVolume; bottomStemVolume4.value = defaultVolume; } }
I haven't been able to make this work for deck3 and 4, and I think that's because of the topDeckId/bottomDeckId.
I'm working on a dynamic code using declarations as follow:
AppProperty { id: topStemVolume1; path: "app.traktor.decks.1.stems.1.volume" }
AppProperty { id: topStemVolume2; path: "app.traktor.decks.1.stems.2.volume" }
AppProperty { id: topStemVolume3; path: "app.traktor.decks.1.stems.3.volume" }
AppProperty { id: topStemVolume4; path: "app.traktor.decks.1.stems.4.volume" }
AppProperty { id: bottomStemVolume1; path: "app.traktor.decks.2.stems.1.volume" }
AppProperty { id: bottomStemVolume2; path: "app.traktor.decks.2.stems.2.volume" }
AppProperty { id: bottomStemVolume3; path: "app.traktor.decks.2.stems.3.volume" }
AppProperty { id: bottomStemVolume4; path: "app.traktor.decks.2.stems.4.volume" }
function updatePropertyPaths() { topStemVolume1.path = `app.traktor.decks.${topDeck}.stems.1.volume`; topStemVolume2.path = `app.traktor.decks.${topDeck}.stems.2.volume`; topStemVolume3.path = `app.traktor.decks.${topDeck}.stems.3.volume`; topStemVolume4.path = `app.traktor.decks.${topDeck}.stems.4.volume`; bottomStemVolume1.path = `app.traktor.decks.${bottomDeck}.stems.1.volume`; bottomStemVolume2.path = `app.traktor.decks.${bottomDeck}.stems.2.volume`; bottomStemVolume3.path = `app.traktor.decks.${bottomDeck}.stems.3.volume`; bottomStemVolume4.path = `app.traktor.decks.${bottomDeck}.stems.4.volume`; // Update Track Type paths topDeckTrackType.path = `app.traktor.decks.${topDeck}.track.content.stem_available`; bottomDeckTrackType.path = `app.traktor.decks.${bottomDeck}.track.content.stem_available`; }
function resetFocusedStemDeckVolumeAndFilter(deckId) { var defaultVolume = 1.0; // Determine if we're working with top or bottom deck var isTopDeck = (deckId === getTopDeckId(decksAssignment)); // Select the appropriate properties based on top/bottom deck var stemVolume = isTopDeck ? [topStemVolume1, topStemVolume2, topStemVolume3, topStemVolume4] : [bottomStemVolume1, bottomStemVolume2, bottomStemVolume3, bottomStemVolume4]; // Reset volume on all channels for (var i = 0; i < 4; i++) { // Set volumes to maximum stemVolume[i].value = defaultVolume; } } enum DecksAssignment { AC, BD } // Add this to trigger reset when a new track is loaded AppProperty { id: deckLoadedProperty; path: "app.traktor.decks." + deckId + ".is_loaded" } onDeckLoadedPropertyChanged: { if (deckLoadedProperty.value) { resetFocusedStemDeckVolumeAndFilter(deckId); } }
Does it look like a promising direction? Or should I look elsewhere?
Thanks a lot for your help!
Comments
-
This is S5/S8/D2 code you are working on, right?
0 -
To the topic creator: Why are you creating two topics?
At first glance it looks as if the S5 has already mastered the functions. (TP4)
AppProperty { path: "app.traktor.decks.1.is_loaded_signal"; onValueChanged: onDeckLoaded(1); } AppProperty { path: "app.traktor.decks.2.is_loaded_signal"; onValueChanged: onDeckLoaded(2); } AppProperty { path: "app.traktor.decks.3.is_loaded_signal"; onValueChanged: onDeckLoaded(3); } AppProperty { path: "app.traktor.decks.4.is_loaded_signal"; onValueChanged: onDeckLoaded(4); } function onDeckLoaded(deckId) { if (deckId == topDeckId || deckId == bottomDeckId) { if (screenViewProp.value == ScreenView.browser) { screenViewProp.value = ScreenView.deck; } } if (stemResetOnLoad && s5mapping.running && hasStemMode((deckId == focusedDeckId) ? focusedDeckType : unfocusedDeckType)) { resetFocusedStemDeckVolumeAndFilter(deckId); } } AppProperty { id: topStemVolume1; path: "app.traktor.decks."+ topDeckId + ".stems.1.volume" } AppProperty { id: topStemVolume2; path: "app.traktor.decks."+ topDeckId + ".stems.2.volume" } AppProperty { id: topStemVolume3; path: "app.traktor.decks."+ topDeckId + ".stems.3.volume" } AppProperty { id: topStemVolume4; path: "app.traktor.decks."+ topDeckId + ".stems.4.volume" } AppProperty { id: topStemFilter1; path: "app.traktor.decks."+ topDeckId + ".stems.1.filter_value" } AppProperty { id: topStemFilter2; path: "app.traktor.decks."+ topDeckId + ".stems.2.filter_value" } AppProperty { id: topStemFilter3; path: "app.traktor.decks."+ topDeckId + ".stems.3.filter_value" } AppProperty { id: topStemFilter4; path: "app.traktor.decks."+ topDeckId + ".stems.4.filter_value" } AppProperty { id: topStemFilterOn1; path: "app.traktor.decks."+ topDeckId + ".stems.1.filter_on" } AppProperty { id: topStemFilterOn2; path: "app.traktor.decks."+ topDeckId + ".stems.2.filter_on" } AppProperty { id: topStemFilterOn3; path: "app.traktor.decks."+ topDeckId + ".stems.3.filter_on" } AppProperty { id: topStemFilterOn4; path: "app.traktor.decks."+ topDeckId + ".stems.4.filter_on" } AppProperty { id: bottomStemVolume1; path: "app.traktor.decks."+ bottomDeckId + ".stems.1.volume" } AppProperty { id: bottomStemVolume2; path: "app.traktor.decks."+ bottomDeckId + ".stems.2.volume" } AppProperty { id: bottomStemVolume3; path: "app.traktor.decks."+ bottomDeckId + ".stems.3.volume" } AppProperty { id: bottomStemVolume4; path: "app.traktor.decks."+ bottomDeckId + ".stems.4.volume" } AppProperty { id: bottomStemFilter1; path: "app.traktor.decks."+ bottomDeckId + ".stems.1.filter_value" } AppProperty { id: bottomStemFilter2; path: "app.traktor.decks."+ bottomDeckId + ".stems.2.filter_value" } AppProperty { id: bottomStemFilter3; path: "app.traktor.decks."+ bottomDeckId + ".stems.3.filter_value" } AppProperty { id: bottomStemFilter4; path: "app.traktor.decks."+ bottomDeckId + ".stems.4.filter_value" } AppProperty { id: bottomStemFilterOn1; path: "app.traktor.decks."+ bottomDeckId + ".stems.1.filter_on" } AppProperty { id: bottomStemFilterOn2; path: "app.traktor.decks."+ bottomDeckId + ".stems.2.filter_on" } AppProperty { id: bottomStemFilterOn3; path: "app.traktor.decks."+ bottomDeckId + ".stems.3.filter_on" } AppProperty { id: bottomStemFilterOn4; path: "app.traktor.decks."+ bottomDeckId + ".stems.4.filter_on" } function resetFocusedStemDeckVolumeAndFilter(deckId) { var defaultVolume = 1.0; var defaultFilter = 0.5; var defaultFilterOn = false; if (deckId == topDeckId) { topStemVolume1.value = defaultVolume; topStemVolume2.value = defaultVolume; topStemVolume3.value = defaultVolume; topStemVolume4.value = defaultVolume; topStemFilter1.value = defaultFilter; topStemFilter2.value = defaultFilter; topStemFilter3.value = defaultFilter; topStemFilter4.value = defaultFilter; topStemFilterOn1.value = defaultFilterOn; topStemFilterOn2.value = defaultFilterOn; topStemFilterOn3.value = defaultFilterOn; topStemFilterOn4.value = defaultFilterOn; } else { bottomStemVolume1.value = defaultVolume; bottomStemVolume2.value = defaultVolume; bottomStemVolume3.value = defaultVolume; bottomStemVolume4.value = defaultVolume; bottomStemFilter1.value = defaultFilter; bottomStemFilter2.value = defaultFilter; bottomStemFilter3.value = defaultFilter; bottomStemFilter4.value = defaultFilter; bottomStemFilterOn1.value = defaultFilterOn; bottomStemFilterOn2.value = defaultFilterOn; bottomStemFilterOn3.value = defaultFilterOn; bottomStemFilterOn4.value = defaultFilterOn; } }
0 -
Yes, I started working for it on my S5, and now I have an S8 so I'd like to make it work for both.
0 -
Ah, sorry, I guessed wrong in making a different thread. It was a different question on the same topic.
Thank you for your input, I'll try that although I'm still on TP3.0 -
Yes, it's best to try it yourself.
If this is not possible, upload your files.
but please don't use any that cause an error.
or read this topic there are some good tips1 -
Hmm, that dynamic approach doesn't work with my S5. The code Claude.ai gave me could load, but the one I got from Pixel crashed on startup.
I'm reverting to my qml that works for deck A and B, good enough for most situations.
Sadly, I tried to add those changes to my S8 qml, but those files are very different and that code is just inactive over there.
It looks like the syntax would be simpler to manage the four decks, but the file structure is so different that I'm not sure how to add my custom qml.
I think that those stem param reset are key to anyone that's using stems seriously, so I hope that someone will figure it out.
0 -
If no one comes forward with an S5 by next weekend, I'll take a look at your file.
edit: Please upload the qml file.
Preferably one without your changes0 -
Well, thank you if you can do something.
Here is the default S5 file:I investigated a little on the S8 side, and it seems that the file is actually in CIS/common/, but I haven't had any success yet.
0 -
I sent you a message😎
1 -
Yes! That code that you shared works both for S5 and S8!
Thanks a lot!
AppProperty { id: keyAdjust; path: "app.traktor.decks." + focusedDeckId + ".track.key.adjust" } AppProperty { id: stemMuted_1; path: "app.traktor.decks." + focusedDeckId + ".stems.1.muted" } AppProperty { id: stemMuted_2; path: "app.traktor.decks." + focusedDeckId + ".stems.2.muted" } AppProperty { id: stemMuted_3; path: "app.traktor.decks." + focusedDeckId + ".stems.3.muted" } AppProperty { id: stemMuted_4; path: "app.traktor.decks." + focusedDeckId + ".stems.4.muted" } AppProperty { id: stemVolume_1; path: "app.traktor.decks." + focusedDeckId + ".stems.1.volume" } AppProperty { id: stemVolume_2; path: "app.traktor.decks." + focusedDeckId + ".stems.2.volume" } AppProperty { id: stemVolume_3; path: "app.traktor.decks." + focusedDeckId + ".stems.3.volume" } AppProperty { id: stemVolume_4; path: "app.traktor.decks." + focusedDeckId + ".stems.4.volume" } AppProperty { id: stemFilterOn_1; path: "app.traktor.decks." + focusedDeckId + ".stems.1.filter_on" } AppProperty { id: stemFilterOn_2; path: "app.traktor.decks." + focusedDeckId + ".stems.2.filter_on" } AppProperty { id: stemFilterOn_3; path: "app.traktor.decks." + focusedDeckId + ".stems.3.filter_on" } AppProperty { id: stemFilterOn_4; path: "app.traktor.decks." + focusedDeckId + ".stems.4.filter_on" } AppProperty { id: stemFilter_1; path: "app.traktor.decks." + focusedDeckId + ".stems.1.filter_value" } AppProperty { id: stemFilter_2; path: "app.traktor.decks." + focusedDeckId + ".stems.2.filter_value" } AppProperty { id: stemFilter_3; path: "app.traktor.decks." + focusedDeckId + ".stems.3.filter_value" } AppProperty { id: stemFilter_4; path: "app.traktor.decks." + focusedDeckId + ".stems.4.filter_value" } AppProperty { id: stemFxSendOn_1; path: "app.traktor.decks." + focusedDeckId + ".stems.1.fx_send_on" } AppProperty { id: stemFxSendOn_2; path: "app.traktor.decks." + focusedDeckId + ".stems.2.fx_send_on" } AppProperty { id: stemFxSendOn_3; path: "app.traktor.decks." + focusedDeckId + ".stems.3.fx_send_on" } AppProperty { id: stemFxSendOn_4; path: "app.traktor.decks." + focusedDeckId + ".stems.4.fx_send_on" } AppProperty { id: stemFxSend_1; path: "app.traktor.decks." + focusedDeckId + ".stems.1.fx_send" } AppProperty { id: stemFxSend_2; path: "app.traktor.decks." + focusedDeckId + ".stems.2.fx_send" } AppProperty { id: stemFxSend_3; path: "app.traktor.decks." + focusedDeckId + ".stems.3.fx_send" } AppProperty { id: stemFxSend_4; path: "app.traktor.decks." + focusedDeckId + ".stems.4.fx_send" } AppProperty { id: deckLoadedSignal; path: "app.traktor.decks." + focusedDeckId + ".is_loaded_signal"; onValueChanged: { if (value) { keyAdjust.value = 0; stemMuted_1.value = false; stemVolume_1.value = 1.0; stemFilterOn_1.value = false; stemFilter_1.value = 0.5; stemFxSendOn_1.value = false; stemFxSend_1.value = 1.0; stemMuted_2.value = false; stemVolume_2.value = 1.0; stemFilterOn_2.value = false; stemFilter_2.value = 0.5; stemFxSendOn_2.value = false; stemFxSend_2.value = 1.0; stemMuted_3.value = false; stemVolume_3.value = 1.0; stemFilterOn_3.value = false; stemFilter_3.value = 0.5; stemFxSendOn_3.value = false; stemFxSend_3.value = 1.0; stemMuted_4.value = false; stemVolume_4.value = 1.0; stemFilterOn_4.value = false; stemFilter_4.value = 0.5; stemFxSendOn_4.value = false; stemFxSend_4.value = 1.0; } } }
1 -
great that I could help🙂
1
Categories
- All Categories
- 18 Welcome
- 1.7K Hangout
- 67 NI News
- 894 Tech Talks
- 4.6K Native Access
- 17.8K Komplete
- 2.2K Komplete General
- 4.8K Komplete Kontrol
- 6.3K Kontakt
- 1.1K Reaktor
- 407 Battery 4
- 917 Guitar Rig & FX
- 465 Massive X & Synths
- 1.5K Other Software & Hardware
- 6.4K Maschine
- 8.2K Traktor
- 8.2K Traktor Software & Hardware
- Check out everything you can do
- Create an account
- See member benefits
- Answer questions
- Ask the community
- See product news
- Connect with creators